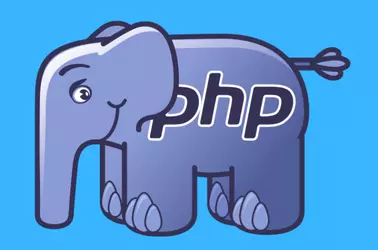
Laravel supported ciphers are AES-128-CBC and AES-256-CBC
On a Laravel application, I get the following error message To fix the error, run the command This will generate
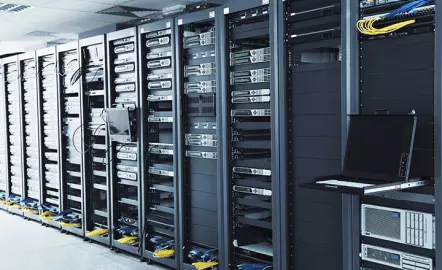
How to clear Laravel cache
To clear laravel cache, run command You can also delete compiled files from
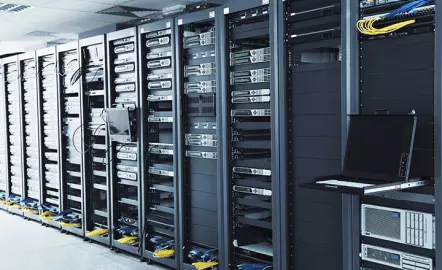
How to find Laravel Framework Version
Laravel is a popular PHP framework. In this post, I will show how to check laravel version. To find Laravel
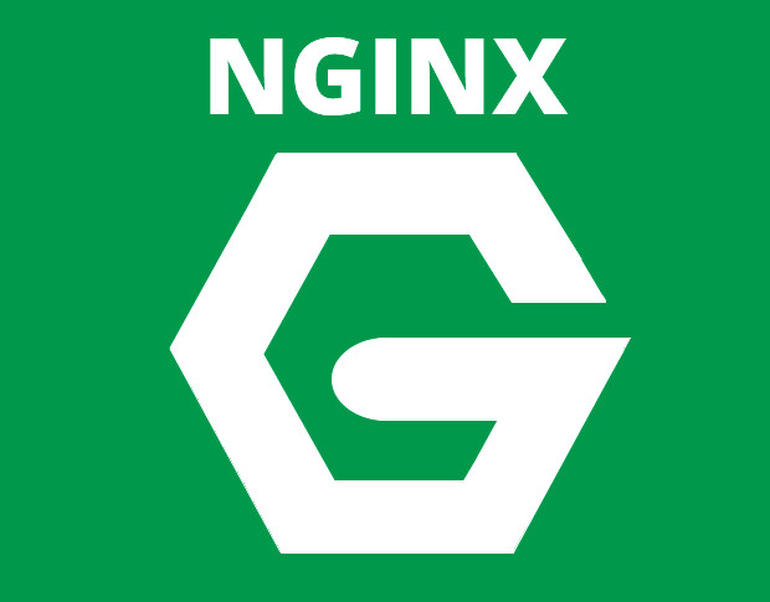
Nginx Config for Laravel Application in sub folder
To run Laravel Application on sub folder of a web site, use following configuration. If you run Laravel application as
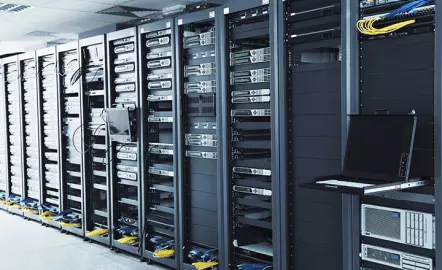
Laravel
Laravel Database Migration Error Key too long How to find Laravel Framework Version How to clear Laravel cache Laravel supported
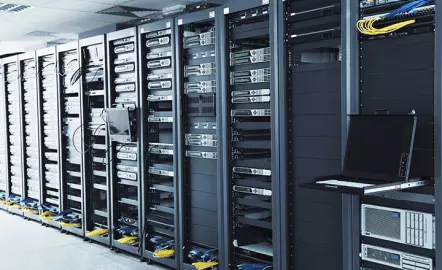
Laravel Database Migration Error Key too long
When doing a database migration on Laravel, i get following error This is because MariaDB use different UTF8 format. To
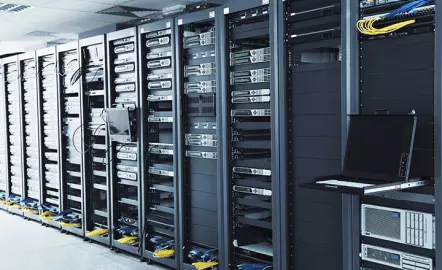
Syntax error or access violation: 1071 Specified key was too long
When running database migration in a new larvel project, i get following error boby@hon-pc-01:~/www/proxy (master)$ php artisan migrate In Connection.php