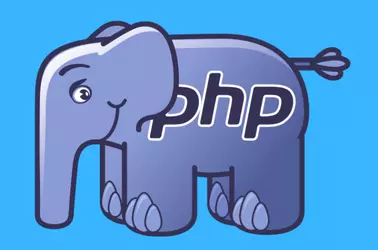
How to get Contents of a Webpage using PHP curl
PHP cURL module allows you to connect to remote websites and API endpoints to exchange data. To see if you
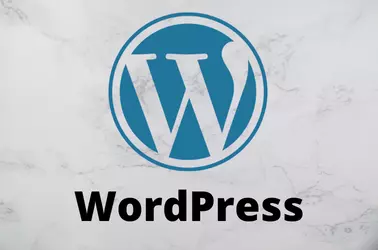
CURL ERROR 7 could not establish a secure connection to WordPress.org
On a WordPress website hosted on a CentOS server, I got the following error on the header of the website.
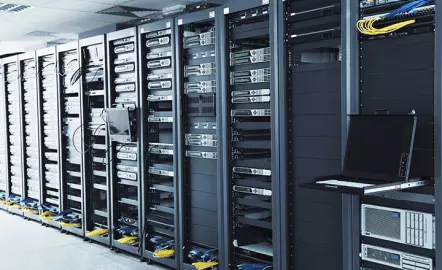
How to use HTTP Basic authentication with cURL
To access sites that use HTTP Basic authentication with curl, use command Or If you don’t specify password, curl will
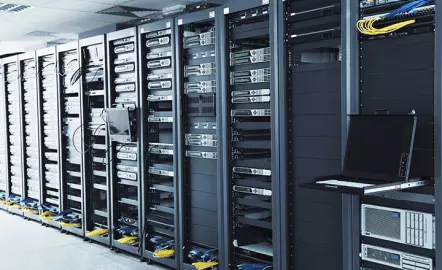
curl using cookie
Here is some example of using curl to login to site, use cookie to do further requests. See curl
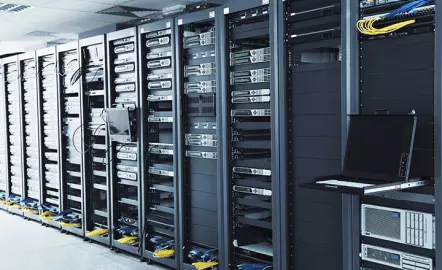
Fetch as Google with curl
A hacked web site got hacked. The site listed on google SERP with text “There are essentially two prescribed drugs
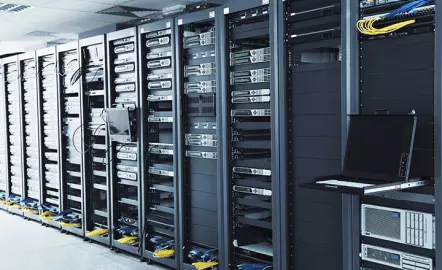
Check if HTTP/2 enabled using curl
To see if a web site have HTTP/2 enabled, you can run If you see 2, the site have HTTP/2
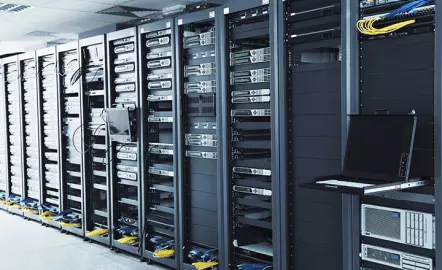
Making POST request from curl
Here is an example POST request using curl Sending JSON string Post a variable
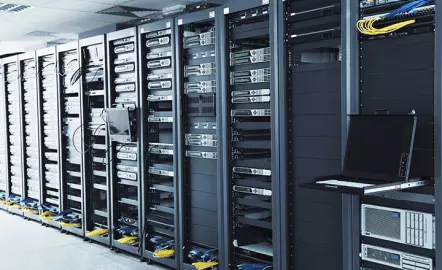
Using Host Header in curl
When you have multiple web site hosted on a server, if you want to access one of the site using
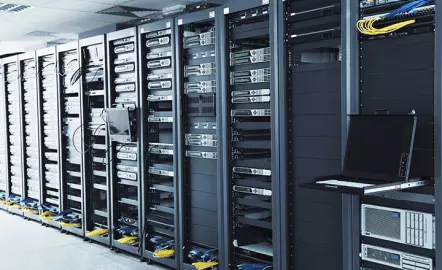
curl
Making POST request from curl Using Host Header in curl curl set user agent Check if HTTP/2 enabled using curl