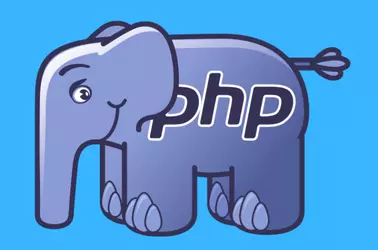
How to install PHP from Source on Ubuntu
Compiling PHP from source code can be essential when you require a specific PHP version that isn’t available through your
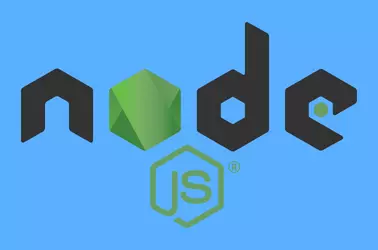
Install Yarn Package Manager
Yarn is a package manager for JavaScript like npm. It is commonly used for managing dependencies for Node.js applications, but
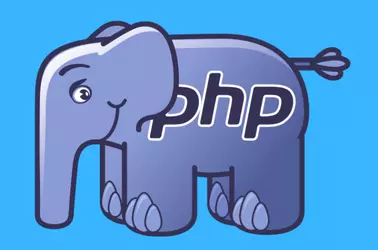
How to get Contents of a Webpage using PHP curl
PHP cURL module allows you to connect to remote websites and API endpoints to exchange data. To see if you
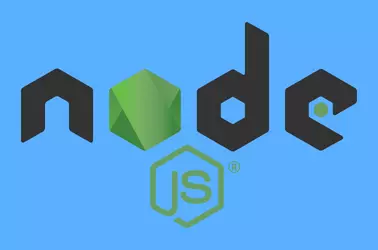
gridsome error:03000086:digital envelope routines::initialization error
When starting a gridsome project on Ubuntu 20.04 with Node.js version v18.10.0, it failed with the error message “error:03000086:digital envelope
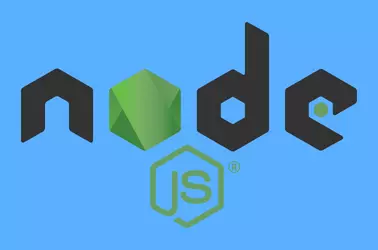
How to change Next.js port
You can start Next.js development server with the command npm run dev This will start the development server on port
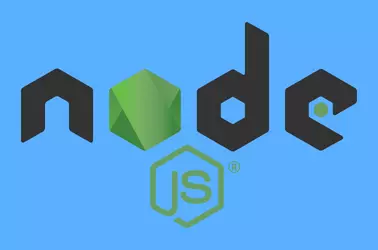
How to Install Yarn Package Manager on Ubuntu
Yarn is a javascript package manager like npm. Import the GPG key curl -sL https://dl.yarnpkg.com/debian/pubkey.gpg | gpg –dearmor | sudo
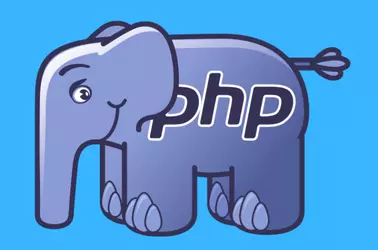
Laravel supported ciphers are AES-128-CBC and AES-256-CBC
On a Laravel application, I get the following error message The only supported ciphers are AES-128-CBC and AES-256-CBC with the
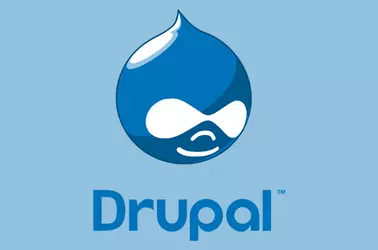
How to change URL of a Drupal site
To change the URL of a Drupal website, edit the file sites/default/settings.php Find the line $base_url = ‘https://www.your-current-domain’; // NO
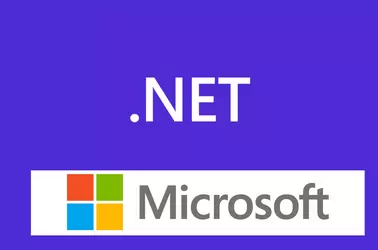
How to create .NET console program
Once you have dotnet SDK installed, you can create your first program. To create a console project run dotnet new
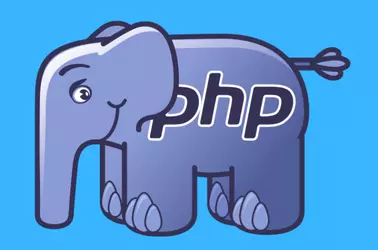
How To Install ionCube on Ubuntu 20.04
ionCube is PHP extension that is used to load ionCube encoded PHP files. ionCube is used to protect commercial PHP
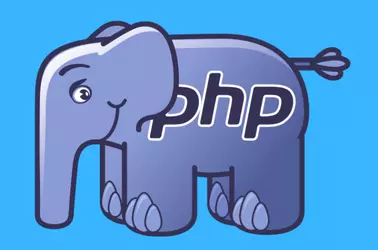
How to install PHP PECL Extension
PECL is a repository for PHP extensions. To install PHP extensions from PCEL, you can use the command pcel install
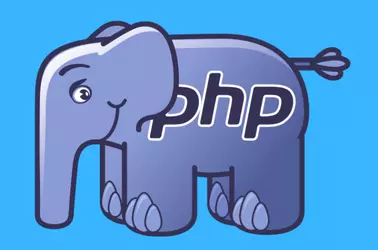
Monitoring APCu with APCu Panel
apcu-panel provide GUI to monitor APCu cache. To install apcu-panel on CentOS, run yum install apcu-panel The package provides following